1.实验目的
通过编写多进程程序,使读者熟练掌握fork()、exec()、wait()和waitpid()等函数的使用,进一步理解在Linux中多进程编程的步骤。
2.实验内容
该实验有3个进程,其中一个为父进程,其余两个是该父进程创建的子进程,其中一个子进程运行“ls -l”指令,另一个子进程在暂停5s后异常退出。父进程先用阻塞方式等待第一个子进程的结束,然后用非阻塞方式等待另一个子进程的退出,待收集到第二个子进程结束的信息后,父进程就返回。
3.实验步骤
(1)画出该实验流程图。该实验流程图如图1所示。
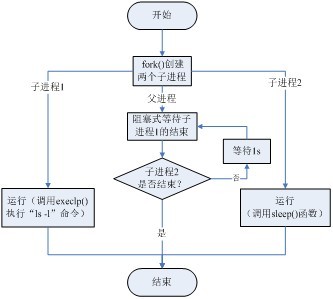 图1 实验流程图
(2)实验源代码。先看一下下面的代码,这个程序能得到我们所希望的结果吗?它的运行会产生几个进程?请读者回忆一下fork()调用的具体过程。
/* multi_proc_wrong.c */
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/wait.h>
int main(void)
{
pid_t child1, child2, child;
/* 创建两个子进程 */
child1 = fork();
child2 = fork();
/* 子进程1的出错处理 */
if (child1 == -1)
{
printf("Child1 fork error\n");
exit(1);
}
/* 在子进程1中调用execlp()函数 */
else if (child1 == 0)
{
printf("In child1: execute 'ls -l'\n");
if (execlp("ls", "ls", "-l", NULL) < 0)
{
printf("Child1 execlp error\n");
}
}
/* 子进程2的出错处理 */
if (child2 == -1)
{
printf("Child2 fork error\n");
exit(1);
}
/* 在子进程2中使其暂停5s */
else if( child2 == 0 )
{
printf("In child2: sleep for 5 seconds and then exit\n");
sleep(5);
exit(0);
}
/* 在父进程中等待两个子进程的退出 */
else
{
printf("In father process:\n");
child = waitpid(child1, NULL, 0); /* 阻塞式等待 */
if (child == child1)
{
printf("Get child1 exit code\n");
}
else
{
printf("Error occured!\n");
}
do
{
child = waitpid(child2, NULL, WNOHANG);/* 非阻塞式等待 */
if (child == 0)
{
printf("The child2 process has not exited!\n");
sleep(1);
}
} while (child == 0);
if (child == child2)
{
printf("Get child2 exit code\n");
}
else
{
printf("Error occured!\n");
}
}
exit(0);
}
编译和运行以上代码,并观察其运行结果。它的结果是我们所希望得到的吗?
看完前面的代码后,再观察下面的代码,比较一下它们之间有什么区别,看看会解决哪些问题。
/* multi_proc.c */
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/wait.h>
int main(void)
{
pid_t child1, child2, child;
/* 创建两个子进程 */
child1 = fork();
/* 子进程1的出错处理 */
if (child1 == -1)
{
printf("Child1 fork error\n");
exit(1);
}
/* 在子进程1中调用execlp()函数 */
else if (child1 == 0)
{
printf("In child1: execute 'ls -l'\n");
if (execlp("ls", "ls", "-l", NULL) < 0)
{
printf("Child1 execlp error\n");
}
}
/* 在父进程中再创建进程2,然后等待两个子进程的退出 */
else
{
child2 = fork();
/* 子进程2的出错处理 */
if (child2 == -1)
{
printf("Child2 fork error\n");
exit(1);
}
/* 在子进程2中使其暂停5s */
else if(child2 == 0)
{
printf("In child2: sleep for 5 seconds and then exit\n");
sleep(5);
exit(0);
}
printf("In father process:\n");
…(以下部分与前面程序的父进程执行部分相同)
}
exit(0);
}
(3)首先在宿主机上编译、调试该程序:
$ gcc multi_proc.c –o multi_proc(或者使用Makefile)
(4)在确保没有编译错误后,使用交叉编译该程序:
$ arm-linux-gcc multi_proc.c –o multi_proc (或者使用Makefile)
(5)将生成的可执行程序下载到目标板上运行。
4.实验结果
在目标板上运行的结果如下(具体内容与各自的系统有关):
$ ./multi_proc
In child1: execute 'ls -l' /* 子进程1的显示, 以下是“ls –l”的运行结果 */
total 28
-rwxr-xr-x 1 david root 232 2008-07-18 04:18 Makefile
-rwxr-xr-x 1 david root 8768 2008-07-20 19:51 multi_proc
-rw-r--r-- 1 david root 1479 2008-07-20 19:51 multi_proc.c
-rw-r--r-- 1 david root 3428 2008-07-20 19:51 multi_proc.o
-rw-r--r-- 1 david root 1463 2008-07-20 18:55 multi_proc_wrong.c
In child2: sleep for 5 seconds and then exit /* 子进程2的显示 */
In father process: /* 以下是父进程显示 */
Get child1 exit code /* 表示子进程1结束(阻塞等待) */
The child2 process has not exited! /* 等待子进程2结束(非阻塞等待) */
The child2 process has not exited!
The child2 process has not exited!
The child2 process has not exited!
The child2 process has not exited!
Get child2 exit code /* 表示子进程2终于结束了*/
本文选自华清远见嵌入式培训教材《从实践中学嵌入式Linux应用程序开发》
热点链接:
1、Linux下多进程编程之exec函数语法及使用实例
2、Linux下多进程编程之fork()函数语法
3、Linux下多进程编程之fork()函数说明
4、Linux守护进程
5、wait()和waitpid()函数
更多新闻>> |